So I bought Gears of War for the PC yesterday and I fired it up, assuming that my PS3 controller would work since it was working nicely on XP pro, but to my surprise, nothing was happening!
After some extensive searching, it turns out that the drivers to make the PS3 Controller work only work on 32 bit OS's. I just came up with a brainstorm and am excited to try it out. I have a feeling none of these user made drivers work because Vista X64 drivers MUST be signed and the drivers are probably not signed.
One way to get around this is to turn driver signing checking off on startup (Just hit F8 when your machine boots). Once that is off, I'll try the drivers again. Maybe it will work this time...
If this doesn't work, I'm tempted to try and create a driver myself. To be honest, I'm a litte afraid of such a task because my C++ kung-fu is not strong. If my original workaround doesn't work, maybe I'll download the SDK's and what-not and see what happens. I will keep you posted on the results.
About Me
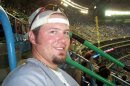
- //Rob
- I'm a C# developer and that takes up most of my time but when I'm not in front of the computer, I do a lot of mountain biking, skiing and spending time with my dog. When I'm not doing any of that stuff, I'm spending time with my lovely wife.
Thursday, September 25, 2008
Friday, September 19, 2008
On Working with Database Images
Last night I ended up having to work with saving and retrieving images from a database and I have found that it's actually very simple to do.
First thing to note - the datatype to used to load and save an image is a byte[].
The next thing you should know is that you cannot run an update or delete statement on an image column without passing the image to the query using a parameter.
To load an image, fill a datatable as you normally would.
The other half of this equation is getting those images saved back to the database which means converting from an image back to a byte[].
So that's how you deal with images in a database. I hope this helps anyone that is having problems with this issue.
First thing to note - the datatype to used to load and save an image is a byte[].
The next thing you should know is that you cannot run an update or delete statement on an image column without passing the image to the query using a parameter.
To load an image, fill a datatable as you normally would.
byte[] imgBytes = (byte[])sqlCommand.ExecuteScalar("Select pic from picTable where pic_id=1",connection);I found most of this code here. Create an System.Drawing.Image object from the resulting byte[].
System.Drawing.Image myImage; System.IO.MemoryStream ms = new System.IO.MemoryStream; // writing the image bytes to the memorystream. ms.write(imgBytes,0,imgBytes.Length); // creating the image from the memorystream. myImage = Image.FromStream(ms,true); ms.Close();You can fill a picturebox or whatever with your shiny new image.
The other half of this equation is getting those images saved back to the database which means converting from an image back to a byte[].
System.IO.MemoryStream ms = new MemoryStream(); // use whatever format you need to here myCurrentImage.Save(ms, ImageFormat.Jpeg); // convert the bytes in the memorystream to a byte[] byte[] imageArray = ms.ToArray();To insert this byte[] into your image column, you'll need to create an SqlParameter object and use that in an SqlCommand object.
Using System.Data.SqlClient; SqlCommand cmd = new SqlCommand("INSERT INTO pic values (@image)"),mySqlConnection); SqlParameter param = new SqlParameter("@image", SqlDbType.Image); param.Value = imageArray;(from above) cmd.Parameters.Add(param); cmd.ExecuteNonQuery();The image should now be saved into your database.
So that's how you deal with images in a database. I hope this helps anyone that is having problems with this issue.
Thursday, September 18, 2008
Transparent Datagridview
The datagridview. I don't like it and it doesn't like me. Today I was trying to get an image to show up behind a datagridview and it was simply not cooperating. The datagridview hates to be transparent for some reason.
Like everything else in my program, I decided to create a custom control. Here's the end result of my labour.
The purpose of this datagridview is to set and show various named locations on a floor plan. Users can select a named location from a combobox and the physical location that it represents will show up on the grid. If the user would like to change that location, they can click on another cell in the datagridview.
To avoid flicker, the style of the control was set to OptimizedDoubleBuffer like this:
To get the background image, I simply created an image property for the grid and set that at design time.
Once that was set, I ovverrode the PaintBackGround method and drew the image to the size of the datagridview. I'm not allowing the user to resize the grid so I won't have to worry about that.
protected override void PaintBackground(Graphics graphics,
I then had to override onMouseClick. Inside that method, if the current cell's background was set to transparent, the Style.BackColor and Style.SelectionBackColor of the current cell would be red, and if the background was red, the Style.BackColor and Style.SelectionBackColor would be set to transparent.
To get the background image to show through the cells, the onCellPainting event had to be overridden. In that event, I painted the background to whatever the current cellstyle is set to.
Here's the entire code. I apologize if it's difficult to read, but formatting code in this blog is very difficult.
Like everything else in my program, I decided to create a custom control. Here's the end result of my labour.
The purpose of this datagridview is to set and show various named locations on a floor plan. Users can select a named location from a combobox and the physical location that it represents will show up on the grid. If the user would like to change that location, they can click on another cell in the datagridview.
To avoid flicker, the style of the control was set to OptimizedDoubleBuffer like this:
SetStyle(ControlStyles.OptimizedDoubleBuffer, true);
To get the background image, I simply created an image property for the grid and set that at design time.
Once that was set, I ovverrode the PaintBackGround method and drew the image to the size of the datagridview. I'm not allowing the user to resize the grid so I won't have to worry about that.
protected override void PaintBackground(Graphics graphics,
Rectangle clipBounds, Rectangle gridBounds) { base.PaintBackground(graphics, clipBounds, gridBounds); graphics.DrawImage(img, new Point(this.RowHeadersWidth, this.ColumnHeadersHeight)); }
I then had to override onMouseClick. Inside that method, if the current cell's background was set to transparent, the Style.BackColor and Style.SelectionBackColor of the current cell would be red, and if the background was red, the Style.BackColor and Style.SelectionBackColor would be set to transparent.
To get the background image to show through the cells, the onCellPainting event had to be overridden. In that event, I painted the background to whatever the current cellstyle is set to.
protected override void OnCellPainting(DataGridViewCellPaintingEventArgs e) { base.OnCellPainting(e); if (e.ColumnIndex > -1 && e.RowIndex > -1) { if (this[e.ColumnIndex, e.RowIndex].Style.BackColor != Color.Red) { this[e.ColumnIndex, e.RowIndex].Style.BackColor = Color.Transparent; } else { this[e.ColumnIndex, e.RowIndex].Style.BackColor = Color.Red; } } }So there you have it. A lovely transparent DataGridView for all.
Here's the entire code. I apologize if it's difficult to read, but formatting code in this blog is very difficult.
using System.Linq; using System.Windows.Forms; using System.Drawing; namespace testproject public class imagedgv : DataGridView { Image img; public Image Img { get { return img; } set {img = value; } } public imagedgv(): base() { SetStyle(ControlStyles.OptimizedDoubleBuffer, true); } protected override void OnSizeChanged(EventArgs e) { base.OnSizeChanged(e); img = new Bitmap(img, this.Width - this.RowHeadersWidth , this.Height - this.ColumnHeadersHeight); } protected override void PaintBackground(Graphics graphics, Rectangle clipBounds, Rectangle gridBounds) { base.PaintBackground(graphics, clipBounds, gridBounds); graphics.DrawImage(img, new Point(this.RowHeadersWidth, this.ColumnHeadersHeight)); } protected override void OnCellPainting(DataGridViewCellPaintingEventArgs e) { base.OnCellPainting(e); if (e.ColumnIndex > -1 && e.RowIndex > -1) { if (this[e.ColumnIndex, e.RowIndex].Style.BackColor != Color.Red) this[e.ColumnIndex, e.RowIndex].Style.BackColor = Color.Transparent; else this[e.ColumnIndex, e.RowIndex].Style.BackColor = Color.Red; } } protected override void OnMouseClick(MouseEventArgs e) { DataGridView.HitTestInfo hti = this.HitTest(e.X, e.Y); if (hti.Type == DataGridViewHitTestType.Cell) { DataGridViewCell c = this[hti.ColumnIndex, hti.RowIndex]; if (c.Style.BackColor == Color.Red) { c.Style.BackColor = Color.Transparent; c.Style.SelectionBackColor = Color.Transparent; } else { c.Style.BackColor = Color.Red; c.Style.SelectionBackColor = Color.Red; } } base.OnMouseClick(e); } }Enjoy!
Wednesday, September 17, 2008
How I Roll
I thought it would be good to post my visual studio theme settings. This was created in VS2008 and can be found here.
Please make sure to save your current visual studio settings before applying this bad boy because it is a dark theme and that may be very different from what you have currently.
If you're looking for a change of pace in how visual studio looks, I found a whole bunch of themes on Scott Hanselman's blog.
That's about it for now, have fun with that theme!
Please make sure to save your current visual studio settings before applying this bad boy because it is a dark theme and that may be very different from what you have currently.
If you're looking for a change of pace in how visual studio looks, I found a whole bunch of themes on Scott Hanselman's blog.
That's about it for now, have fun with that theme!
Hello World!
I've been doing a lot of extra developing lately outside my 9-5 and have come across new issues to overcome almost daily. I thought it would be nice to share my solutions with everyone so that you people won't have to suffer through the tears and rage these things can induce. :)
I sure hope some of this code will be useful to some of you.
Thanks for reading!
P.S. I've included a nifty C# to VB converter because I know a lot of you use VB as your weapon of choice.
I sure hope some of this code will be useful to some of you.
Thanks for reading!
P.S. I've included a nifty C# to VB converter because I know a lot of you use VB as your weapon of choice.
Subscribe to:
Posts (Atom)